Exception Handling
Problems are going to happen in any aspect of life. They may be complex or as simple as not having any coffee left in your house when you wake up. You need your morning caffeine so you decide that you can either go to the coffee shop and get a cup for that morning or you can go to the store and buy more coffee beans so that you can keep making coffee every morning. Either way, you have a solution and a way to handle the problem.
This is no different for software developers when they encounter exceptions. What is an exception and how is it handled? Exceptions are just a type of error that occurs during the execution of a program.
Exceptions are going to happen. They are inevitable. Newer developers or developers fresh out of school may not know how to deal with exceptions or may not know the best ways to manage them. This blog is meant to describe how to handle exceptions properly.
How To Manage Exceptions
Rather than describing what the diverse types of exceptions are and what they mean (that’s what StackOverflow is for), we will discuss how to handle exceptions to not disrupt the application when it is running. We will give a couple of examples as well.
The basic exception handling logic is as follows: Try -> Catch -> Finally, and Throw. This is done in blocks. If you’re not sure what the block is, don’t worry, we will show an example later. Here is a brief description of the exception-handling logic:
- Try – a block that is used to encapsulate an area of code. Errors thrown within the Try block of code will be redirected to the Catch block. This will avoid disrupting the execution of the program.
- Catch – this block of code is executed whenever an exception occurs in the related Try block. Here, the exception can be managed, logged, or ignored.
- Finally – certain code can be placed in a Finally block that will be executed whether an exception occurs. This is an optional block when handling exceptions.
- Throw – is a keyword and not a block of code. The Throw keyword can be used to manually create an exception when it occurs in any of the above Exception Handling Blocks.
Let’s look at a basic example of a Try-Catch block in c#:
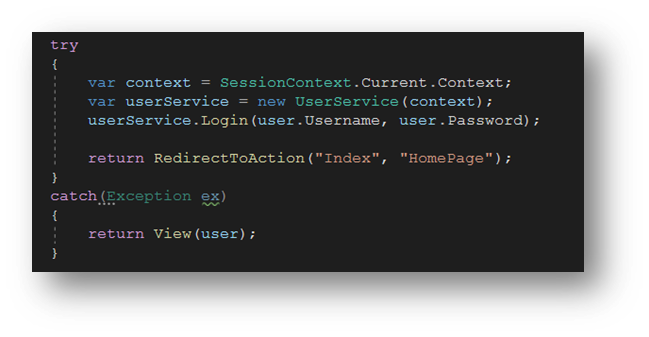
Here, we have a session context created, a database context, and an attempted Login action using a previously defined user object called, “User”.
As you can see, this code is wrapped in a Try block. The Try block will execute the code that is placed inside it and if any exceptions are thrown, the execution of the code will be redirected to the Catch block.
Once the thrown exception is caught by the Catch block, the exception can be handled. In the example given, the code is redirected to the login page view. This is a simple example to demonstrate what the Try-Catch block does. We can see that there is a variable of type Exception called “ex” and this will display the exception that is thrown.
Exceptions can and should be logged either in a database log table or in a plain text file, as shown below with a simple example:
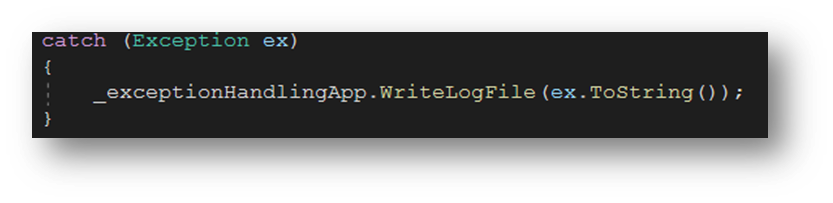
Here we have the exception being caught and written to a text file via an internal function defined as “WriteLogFile”. This function takes in any string and writes it to a text file where the application is located.
Logging exceptions makes debugging and resolving any bugs easier, should any arise because the exception information will be stored and can provide useful details as to why the exception was thrown in the first place and about where the exception was thrown in the code.
After exceptions are caught and handled using the Try-Catch method, you can continue the code and deal with any variables that may need to be cleaned up. This is where the Finally block is used and is completely optional.
In this example, we can see that any exceptions that may occur when either opening the SQL connection or loading the table information will be caught but ignored (there is no code located in the Catch block to log or handle the exceptions), and the SQL connection that was previously established is closed out. This will happen no matter if an exception occurs or not, since this code is placed inside the Finally block.
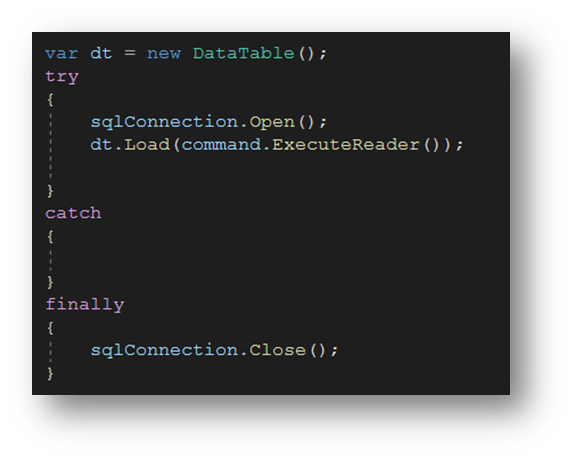
Lastly, let us look at the Throw keyword. This is used to throw exceptions, including user-defined exceptions whenever one occurs. I’ve changed the first Try-Catch example provided to show how a user-defined error can be thrown with the “Throw” keyword:
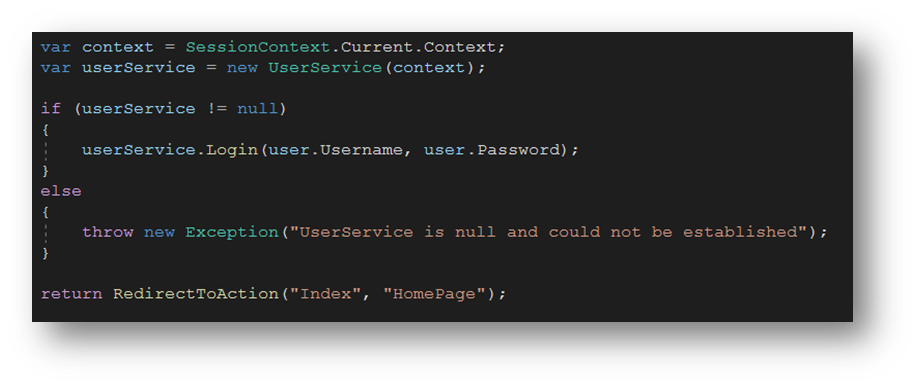
You can see that the Login action is still attempted after a null check of the UserService object. If the object is null, then the Throw keyword is used to throw the exception defined. Defined exceptions must be in the form of a string. You can see my custom exception, “UserService is null and could not be established”.
Here the Throw keyword is not being used in a Try-Catch block, which can be done, but I would recommend always using the Try-Catch method so that the program is not interrupted. The Throw keyword can be extremely helpful at pinpointing the specific area in code an exception is thrown, especially when paired with a Try-Catch block.
Error handling is usually a topic that is not covered enough for developers who are still in school or early in their careers. It is something that is picked up along the way and the hope is that you get better at it. This is the wrong approach though.
Exceptions are going to happen. This should be a topic that is covered more often. Students should know and understand exception handling before they get their first real gig.
Hopefully, this blog will help newer developers to understand the basics of exceptions and how to handle them.
If you’d like to consult with one of our many experienced developers, get in touch with us!