This article showcases a native mobile app built with the Flutter SDK to consume Liferay web services. The native mobile app displays a list of Liferay blogs and allows you to browse and read ones you like. If you’ve never heard of Flutter, it is a relatively new mobile application development SDK created by Google and has gained a lot of traction recently, and for good reason. This cross-platform mobile SDK helps create beautiful mobile UI and enhances developer productivity.
Most organizations want their mobile application user experience to be incredible, but that often comes with a hefty price tag because you have to maintain two separate code bases; one for Android and another for iOS. Flutter allows you to build native apps on iOS and Android from a single codebase. This can be an attractive proposition for applications with the two separate codebases, as well as other hybrid mobile applications where the user experience leaves some things to be desired.
Here is an example of what a simple Flutter native mobile web app may look like consuming Liferay Blogs over RESTful web services :
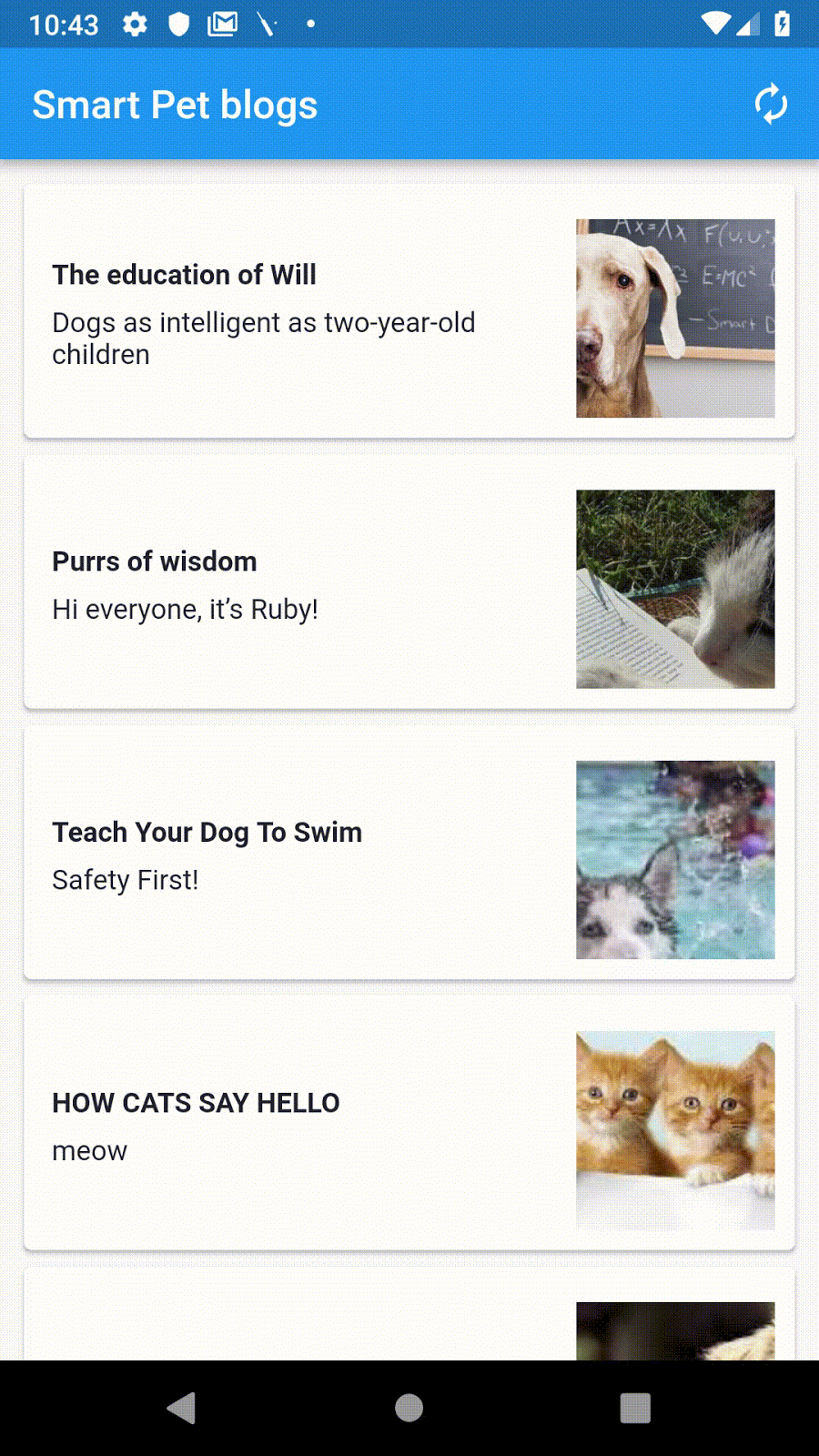
Liferay Setup
We created a few blogs in Liferay DXP 7.1 that we wanted to display in a native mobile app using Flutter. Our RESTful web services provide the methods for reading 1) a list of blogs, and 2) the details of a specific blog. The methods in our component call Liferay’s blog service, select/transform the necessary data into model objects and serialize that in JSON format.
The component declaration is as follows:
@Component(
property = {
JaxrsWhiteboardConstants.JAX_RS_APPLICATION_BASE + "=/blogs",
JaxrsWhiteboardConstants.JAX_RS_NAME + "=Blogs.Rest"
},
service = Application.class)
public class BlogsRestApplication extends Application {
@GET
@Path("/all")
@Produces(MediaType.APPLICATION_JSON)
public String getBlogs() {
...
}
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public String getBlogDetail(
@PathParam("id") long blogId) {
...
}
...
}
OAuth2 Configuration
Liferay DXP 7.1 requires the web service authorization by default. For that, we need to create and configure an OAuth2 application to grant access to our web service. Fortunately, Liferay DXP 7.1 makes this job easy by providing a clear set of instructions. For this app, we used the OAuth2 “Client Credentials” grant type to obtain an access token to authorize the mobile app access to the Liferay web services. In other words, we use Client ID and Client Secret in the Flutter mobile app and configure Liferay to authorize our native mobile app users to see Liferay’s blogs.
Under control panel->Configuration->OAuth Administration, our configuration looked like :
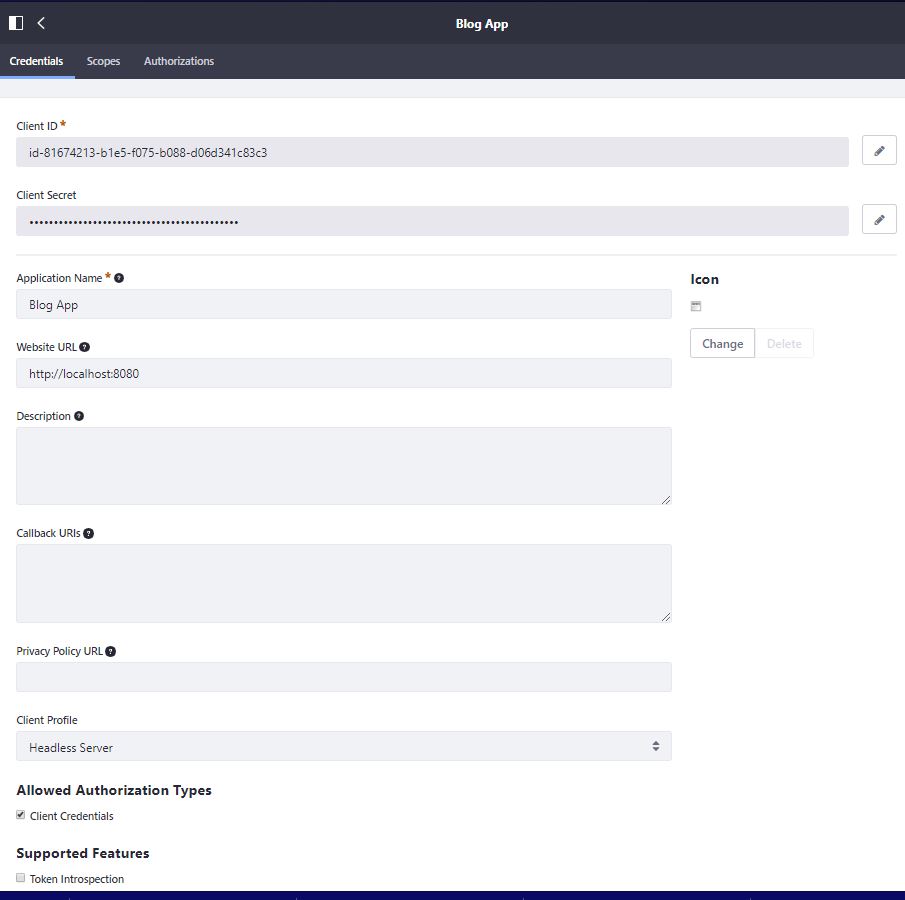
The scope of access only allows GET requests as follows:
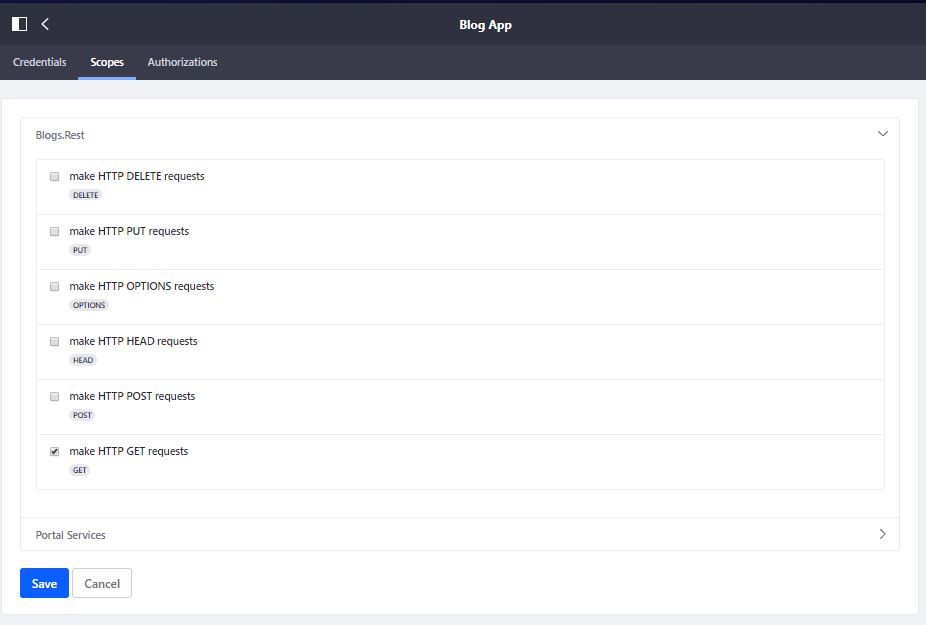
You can test the OAuth2 functionality using a simple curl:
C:\Users\xxxxxxx>curl http://localhost:8080/o/oauth2/token -d "grant_type=client_credentials&client_id=id-81674213-b1e5-f075-b088-d06d341c83c3&client_secret=secret-f96fc8f3-1c92-92b1-b86c-ed171230408f"
{"access_token":"61cda467758a9c1060d723b2a69dc3758223c2bb04aa487d6ba73e609958b6","token_type":"Bearer","expires_in":600,"scope":"GET"}
This gives us the access token and how long it’s valid for. We tested the web service using this token:
C:\Users\xxxxxxx>curl --header "Authorization: Bearer 61cda467758a9c1060d723b2a69dc3758223c2bb04aa487d6ba73e609958b6" http://localhost:8080/o/blogs/all
{"javaClass":"java.util.ArrayList","list":[{"createdDate":"20190121","javaClass":"org.chetan.blogs.rest.application.model.MyBlog","imageUrl":"http://localhost:8080/documents/20126/35991/dog1.jpg/0240fa14-8e53-2d0e-a693-429457843ecf?t=1548108543837","id":35984,"title":"Hello Blog","content":"Woof"}]}
Flutter Native Mobile App
There are many reasons why we picked flutter (eg. 5 reasons why you may love Flutter or Whats revolutionary about Flutter ) for this blog.
Flutter is a mobile development SDK brought to you by Google to help you build fast, beautiful, and cross-platform native mobile applications. It uses a new language called Dart. If you are worried about having to learn a whole new programming language to build Flutter Apps, don’t be! Dart is similar to languages like JavaScript and Java. This helps to reduce the learning curve significantly.
One key advantage of Flutter is that it provides reactive views without the need for JavaScript Bridge, hence improving application performance on the mobile device significantly. Another advantage is the use of widgets. In Flutter, all visual building blocks are Widgets.
Basic knowledge of Flutter is helpful to understand this part. The entire Flutter project is available here.
Screens
- Blogs List
- Blog Detail
We got the OAuth 2.0 access token as follows:
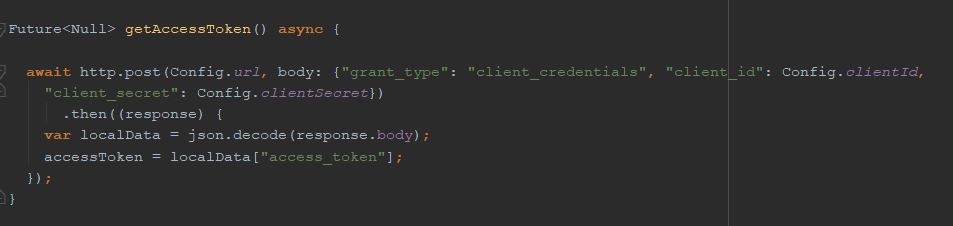
We also used a Card widget inside a ListView widget to display the blogs from Liferay DXP 7.1 web service.
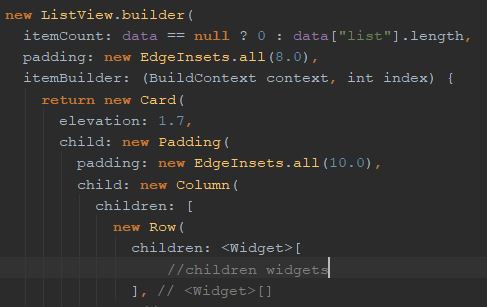
Finally, we handled the time-sensitive nature of the token as follows:
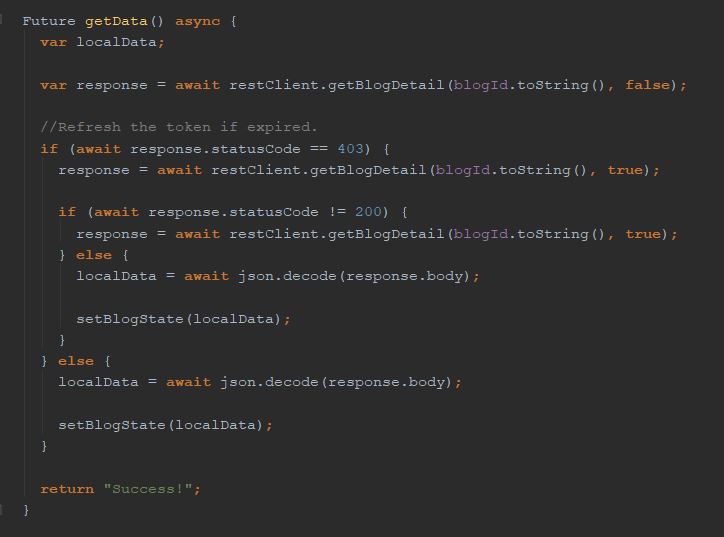
Summary
You can use Flutter to build native mobile apps that consume Liferay DXP web services. With increased performance, a potentially better user experience than a hybrid mobile app approach, and flexibility with your existing Liferay DXP implementation, it’s worth doing a proof of concept for your business. Here are some of our key takeaways:
- Flutter is a mobile SDK based on Dart language. Dart has similarities with Java and JavaScript, so the learning curve is not high.
- There is a lot of built-in widgets that help with developer productivity. Flutter codelabs are a great place to start learning.
- Both Android Studio and Visual Studio Code have excellent support for coding Flutter applications.
- There are a lot of articles on Flutter to help you get going.
If you have questions on how you can best use Flutter for your mobile app or need help with your Liferay DXP implementation, please engage with us via comments on this blog post, or reach out to us.
Additional Reading
You can also continue to explore Flutter and Liferay DXP by checking out Starting out with Flutter, Learning Flutter or The Top 10 New Features in Liferay DXP in the Liferay 7 Knowledge Base.